In this Post, Difference Between Properties and Variables in C#: Explained, We will explain the Difference Between Properties and Variables in C#.
When developing applications in C#, understanding the distinction between properties and variables is crucial for writing clean, maintainable, and efficient code.
Though both are used to store data, they have different characteristics and serve distinct purposes.
Variables in C#
Variables are fields that store data directly and are accessed directly. They do not inherently provide encapsulation.
In C#, a variable is declared with a specific type followed by its name. For example:
public class Person
{
public string name; // Variable
}
This example shows a public variable name
in a class Person
.
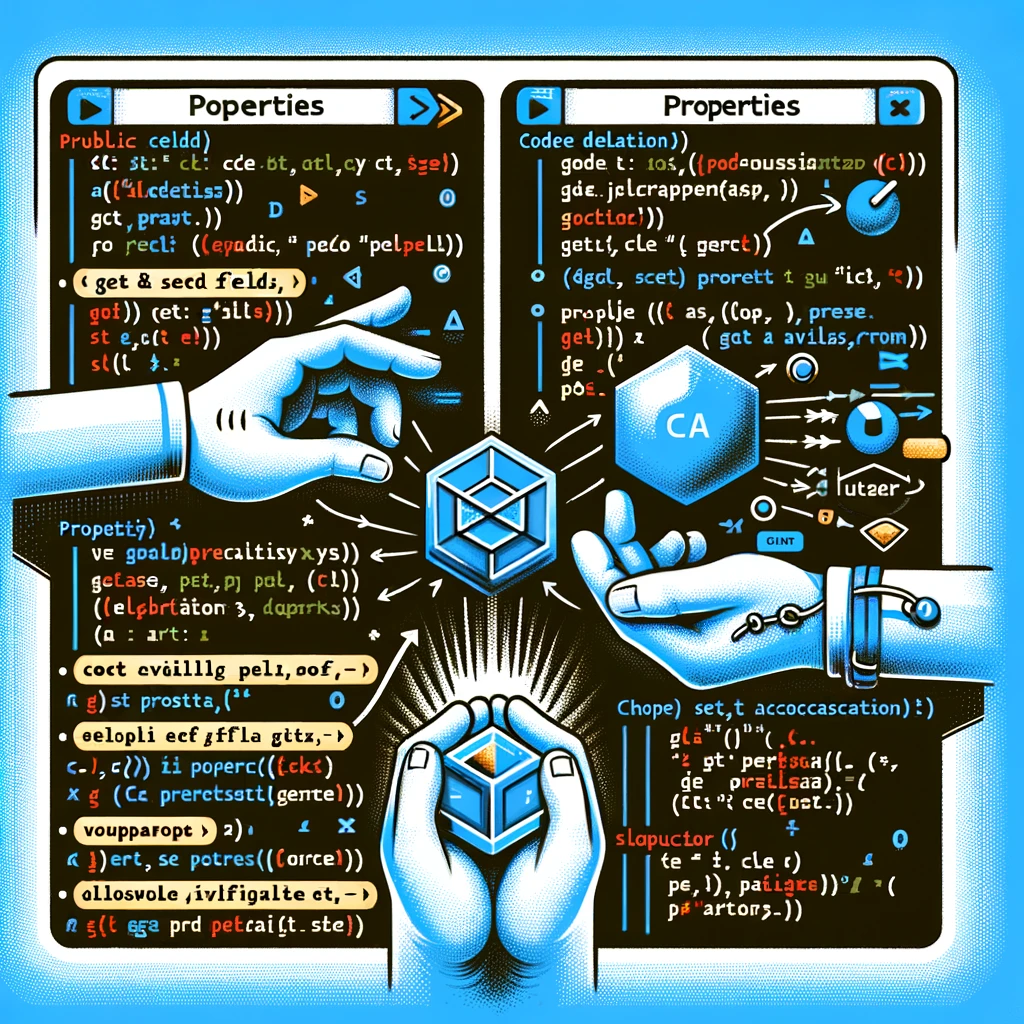
Difference Between Properties and Variables in C#: Explained
This approach is straightforward but it lacks control over how the variable is accessed or modified, making it unsuitable for scenarios that require validation or specific access control.
Properties in C#
Properties, on the other hand, provide a flexible mechanism to read, write, or compute the value of a private field.
They encapsulate the data, allowing validation, logging, or other processing when getting or setting values. Properties are declared using get and set accessors:
public class Person
{
private string name; // Private variable (field)
public string Name // Property
{
get { return name; }
set { name = value; }
}
}
In this example, the Name
property controls access to the private name
field.
Properties can have different access levels for the get and set accessors, allowing scenarios like read-only or write-only properties.
Key Differences
- Encapsulation and Validation: Properties can encapsulate the internal representation and provide validation logic, whereas variables cannot.
- Access Control: Properties allow different access levels for get and set, offering more control over how data is accessed and modified.
- Computed Values: Properties can dynamically compute values, while variables simply hold values.
Example with Validation
public class Person
{
private int age; // Private variable
public int Age // Property with validation
{
get { return age; }
set
{
if (value < 0 || value > 120)
throw new ArgumentOutOfRangeException("Age must be between 0 and 120.");
age = value;
}
}
}
In this example, the Age
property includes validation logic to ensure the age value is within a reasonable range, something a simple variable cannot do.
Conclusion
Understanding when to use properties and when to use variables is essential for robust C# programming.
Use variables for simple, internal storage where direct access is acceptable, and properties for public interfaces that require encapsulation, validation, or computed values. This distinction helps in writing cleaner, more maintainable code.